Let’s use Python to find out which TCP ports are open on your machine and identify possible services that are running on the machine.
We often have doubts about which ports are being used to wait for an incoming connection on a machine. The ports that are waiting for connection are in a LISTEN state and therefore waiting to receive TCP segments with the SYN flag.
After receiving a TCP segment with the SYN flag, the application that has a port open in LISTEN mode responds with a TCP segment with the SYN+ACK flags (second stage of the three way handshake).
Python: How to check my TCP ports?
To make this program we will need to install the psutil library.
The psutil library can be used on Windows, Linux and macOS.
In this post we will use the psutil library on Linux Ubuntu. Let’s use the command below to first install pip to manage the psutil download.
sudo apt install python3-pip
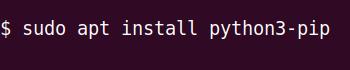
Installing the python psutil library
Next we will install psutil with pip. This library is very interesting for making a series of measurements ranging from memory to network connections.
To install psutil, we will use the command below.
pip install psutil
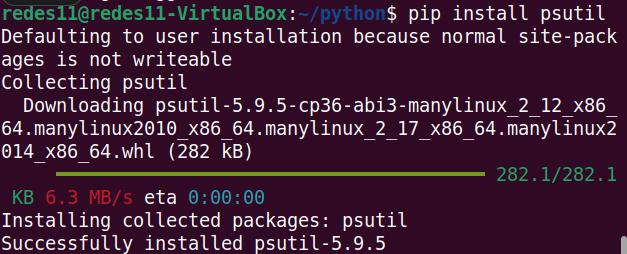
Writing the program to show TCP ports
Now, let’s create a file with the editor of your choice with the name “myports.py”.
nano myports.py
Next, let’s take the text below and paste it into the file myports.py.
The file has comments that help you understand each step.
#-----------------------------------------------------------------------
#Let's import psutil library
import psutil
# Get all my connections
connections = psutil.net_connections(kind='inet')
# filter to get only ports equal to LISTEN
my_ports = [conn.laddr.port for conn in connections if conn.status == psutil.CONN_LISTEN]
# Exclude duplicate ports
my_ports = list(set(my_ports))
# Order from smallest to largest port
my_ports.sort()
# Show the TCP ports that is waiting for connection (LISTENING)
for port in my_ports:
print(f"My Open TCP port= {port} is LISTENING for TCP connection")
#-----------------------------------------------------------------------
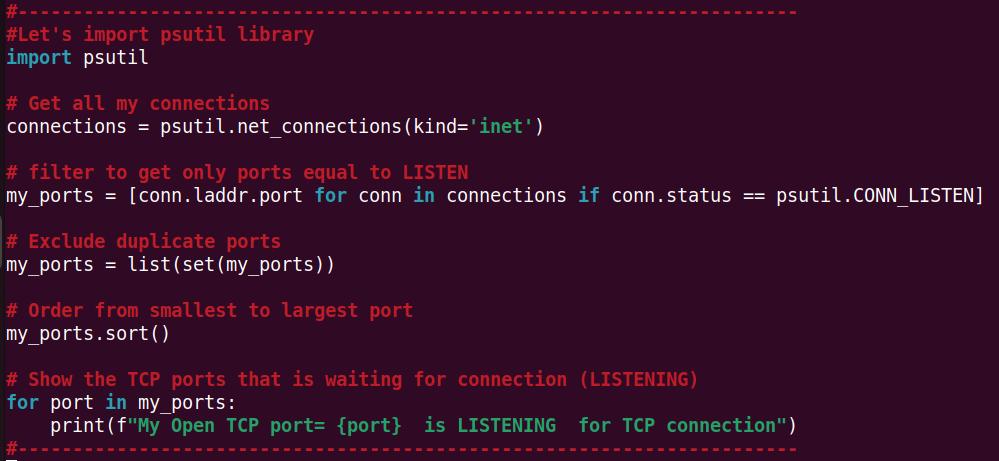
After creating the program to show the local TCP ports that are open, let’s run the python program with the command below.
python3 myports.py
Next, we will see a result like the one shown below.
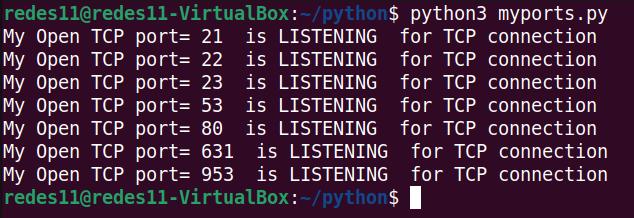
We can see in the figure above that the program showed the TCP ports that are open in listening mode.
Checking the result with netstat
One way to check if the program we created in Python is correct is to use the “netstat -nlt” command. This command allows us to see the open ports on the local machine.
netstat -nlt
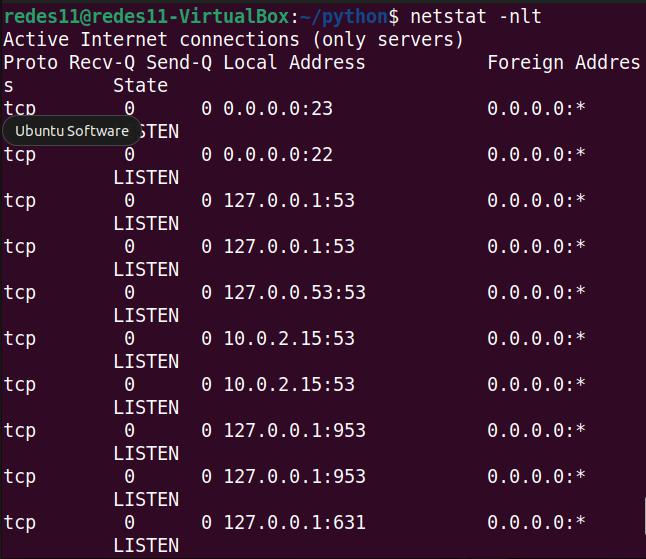
We can see in the figure above that the same open ports that were found with our Python program are also the same ports that the netstat -nlt command found.
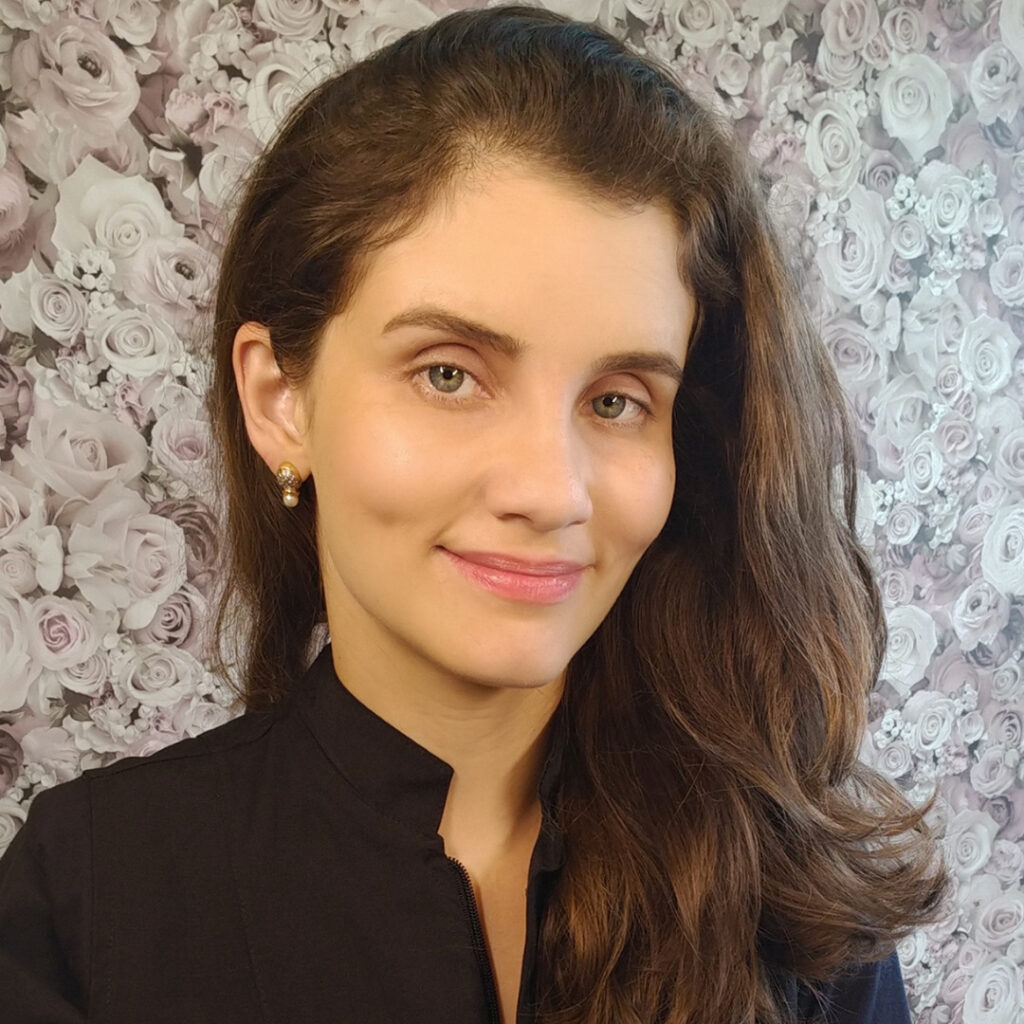
Juliana Mascarenhas
Data Scientist and Master in Computer Modeling by LNCC.
Computer Engineer
See more: