Let’s suppose we want to find out which TCP and UDP ports are open on our computer. This is important because these ports are waiting for communications.
When your ports are in this state it means they are ready to receive messages from other places.
A brief explanation of how TCP and UDP ports work
When another machine tries to connect to ours, it can send a special message called SYN to one of our TCP ports. This way, if one of our ports is open and ready for connection, we respond with a special message called SYN+ACK, showing that we are ready to talk.
This is like a “handshake” between computers before they start exchanging information. This conversation between the doors has 3 steps and is called a “three way handshake”.
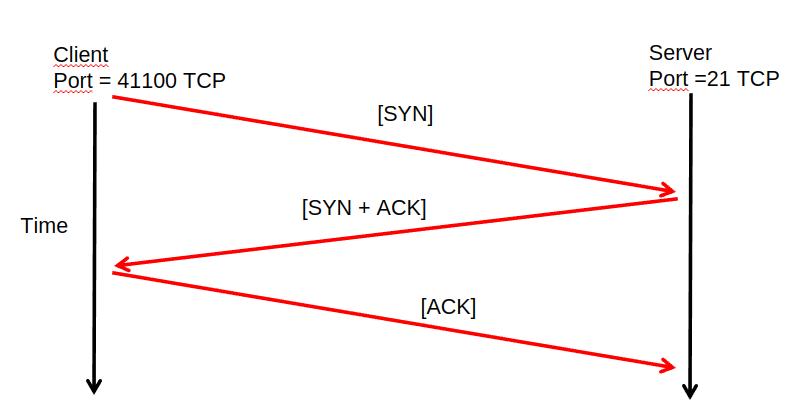
How to show UDP ports in python?
For UDP ports, we do not have an initial connection. Therefore, open UDP ports wait for UDP segments to be sent directly without any initial “handshake.”
It is worth mentioning that when we use we are sending data to an open UDP port, we have two possibilities: The first is that the destination’s UDP port is open and consequently accepts the data we send.
And the other possibility is that the destination port is closed and consequently the destination would not receive the data.
So, using Python, we can find out our open ports and see what services (things that run on the computer, like a web server) are waiting to talk to other computers.
It’s a way to understand what’s happening on the machine and which programs are ready to communicate with others over the internet.
Installing python libraries?
To make our program, we need to install a special tool called “psutil library”. This library is useful because it helps us do lots of cool things with computers that use Windows, Linux or macOS systems.
In our example, we will use Linux Ubuntu. First, let’s install a tool called “pip“. pip is a way to easily download and install other tools, like psutil.
To install pip, let’s open a terminal and type the command below.
sudo apt install python3-pip
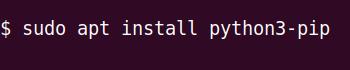
Installing the python psutil library
After that, we will use pip to install psutil. psutil is a Python library that helps us do many interesting things with the computer!
To install psutil, open the terminal and type the command below.
pip install psutil
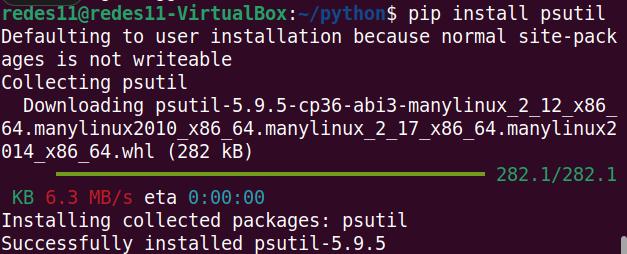
Note. In our code, we will also use the socket library that comes installed in the most of the time with python. Therefore, we do not need to install this library.
The python code to show our TCP and UDP ports
Now, let’s create a file with the editor of your choice with the name “myports_tcp_udp.py”.
nano myports_tcp_udp.py
After that, copy the following text and paste it into the file called myports_tcp_udp.py. This file has comments that explain each step of the code, which will help you better understand what is happening.
import psutil
import socket
# collecting information about TCP and UDP
connectall = psutil.net_connections(kind='inet')
only_udp = [conn for conn in psutil.net_connections(kind='inet') if conn.type == socket.SOCK_DGRAM]
# Separating information about TCP ports
only_tcp_listening_ports = [conn.laddr.port for conn in connectall if conn.status == psutil.CONN_LISTEN]
# Separating information about UDP ports
only_udp_listening_ports = [conn.laddr.port for conn in only_udp]
# removing repeated ports from the list
only_tcp_listening_ports = list(set(only_tcp_listening_ports))
only_udp_listening_ports = list(set(only_udp_listening_ports))
# arranging the ports from smallest to largest
only_tcp_listening_ports.sort()
only_udp_listening_ports.sort()
# Showing only TCP ports
for port in only_tcp_listening_ports:
print(f"TCP port = {port} Open")
print("\n")
#Showing only UDP ports
for port in only_udp_listening_ports:
print(f"UDP port = {port} Open")
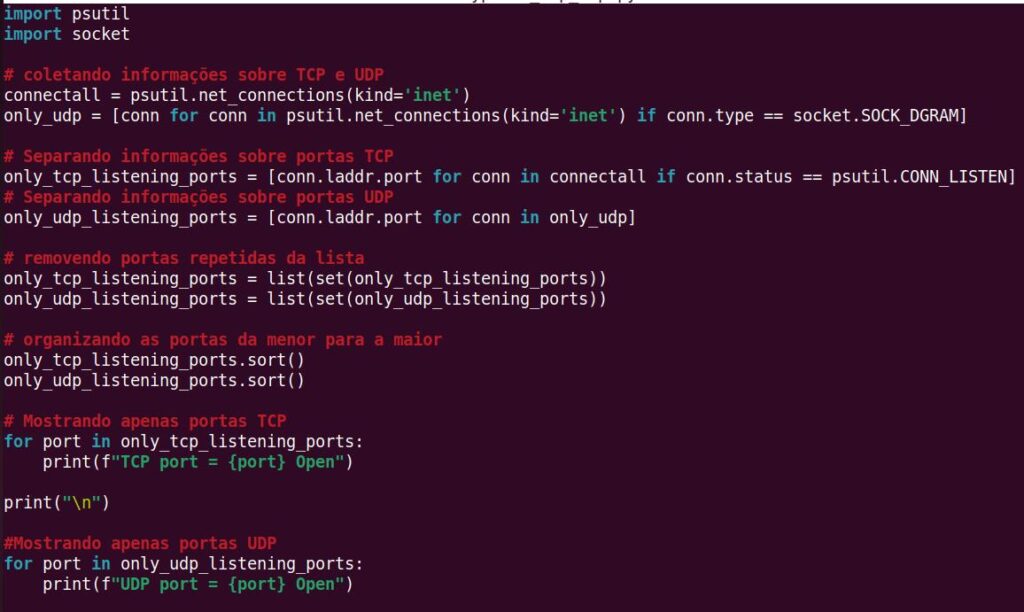
Running the program to show TCP and UDP ports
After creating the program to show the local TCP ports that are open, let’s run the python program with the command below.
python3 myports_tcp_udp.py
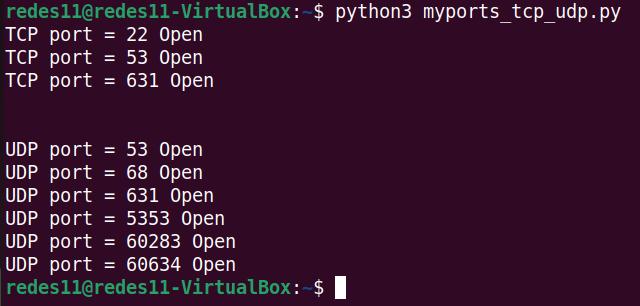
We can see in the figure above that the program showed the TCP and UDP ports that are open on our machine.
Checking our python program
One way to check if the program we created in Python is correct is to use the “netstat -nlt” command. This command allows us to see the open ports on the local machine.
netstat -nltu
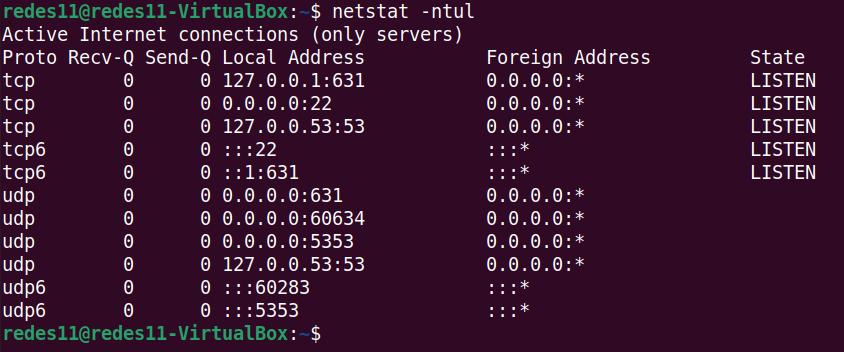
We can see in the figure above that the same open ports that were found with our Python program are also the same ports that the “netstat -ntul” command found .
How to install netstat
If you are on a Linux machine without netstat, you can install it with the command below.
sudo apt install net-tools
And then use the command below.
netstat -nltu
Or you can use the command below.
ss -ntul
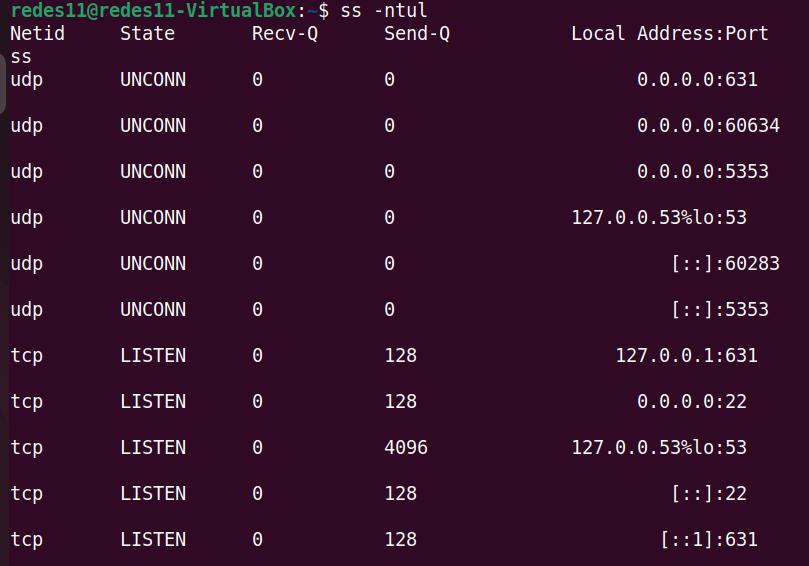
In this case, using the ss command we can also see the same ports that our Python program showed.
See more:
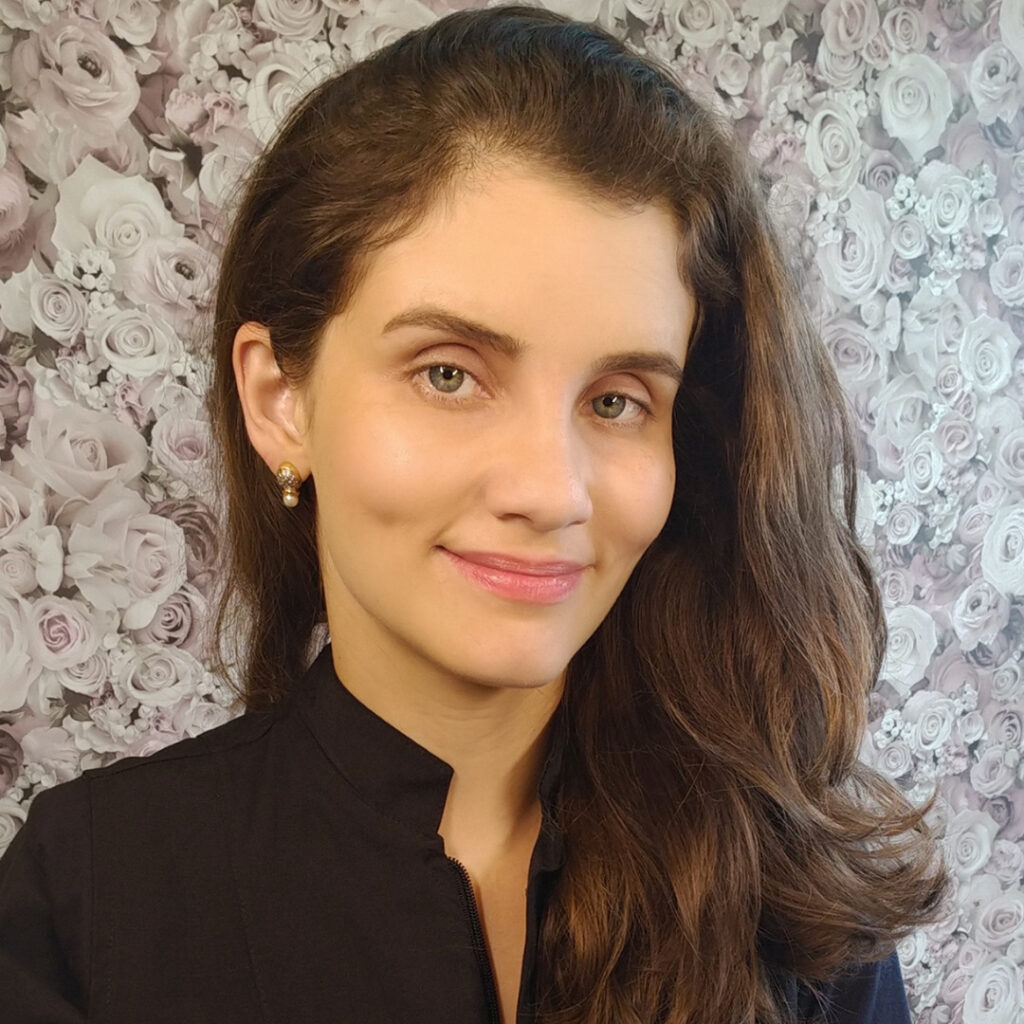
Juliana Mascarenhas
Data Scientist and Master in Computer Modeling by LNCC.
Computer Engineer
Python get metadata from images and pdfs
How to use Ngrok
Install ubuntu 24 on virtualbox
How to X11 Forwarding using SSH
How to handle PDF in Python?
How to install Ubuntu Server on VirtualBox
More links:
https://www.cisco.com/c/en/us/support/docs/ip/routing-information-protocol-rip/13769-5.html