Tutorial for creating a simple chat using UDP sockets in Python 3. The goal is to demonstrate unidirectional communication, where a client sends messages to a server.
Learning to use sockets in python is a great way to combine programming knowledge and networks.
Creating the chat in UDP.
First we will show you how to create a chat using UDP. The best part of UDP is its simplicity and speed to transmit information.
However, UDP does not treat errors or sort the segments, this can lead to some errors in applications such as chat and file transfer.
If you want to create a TCP socket chat click here.
Explaining the UDP chat server.
Now, let’s show the chat server code in python. Then we will show you how to execute the code and then we will explain the code.
#!/usr/bin/python3
# server_UDP.py
import socket
# Request the port that the server should listen on
server_port = int(input("Enter the port the server should listen on: "))
server_ip = '' # Server IP address; '' means it will listen on all interfaces
# Create the UDP socket
udp = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# Define the server address (IP and port)
server_address = (server_ip, server_port)
udp.bind(server_address) # Bind the IP and port to start listening
print(f"Server listening on port {server_port}...")
try:
while True:
# Receive data from the client (data, address)
received_message, client_address = udp.recvfrom(1024)
# Decode the message from bytes to string
received_message = received_message.decode("utf8")
print(f"Received '{received_message}' from {client_address}")
except KeyboardInterrupt:
print("\nServer shutting down.")
except Exception as e:
print(f"An error occurred: {e}")
finally:
udp.close()
Running the UDP socket server on Windows.
Now we will open a Windows editor. In this case, we are using the Notepad to paste the code we copy. After paster the code, click “save as“.
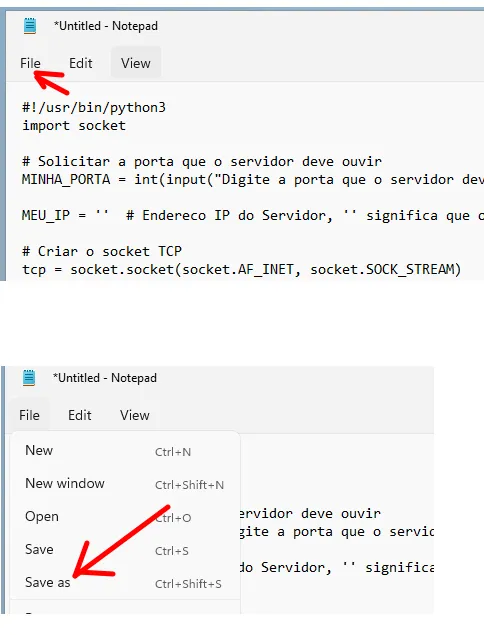
Next, we will give the name “serverudp.py” and we will click save. Remember where you are saving the file.
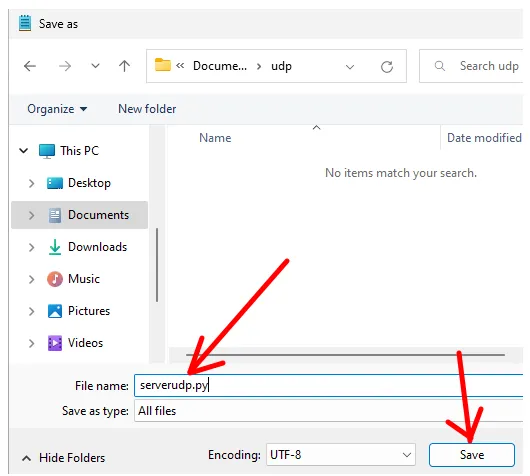
Now, we go where you saved the file “serverudp.py” and let’s double click with the mouse to execute it. After running the “serverudp.py” we will choose the port that the server will listen.
In that case, we’re making the server listen on port 4040.
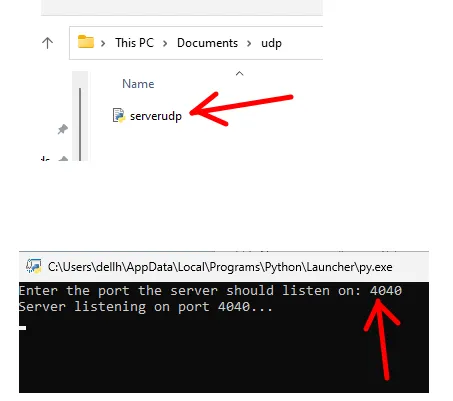
If necessary, allow access to Windows Defender Firewall. In this case, you can choose between access for private network only or access to public networks. See which access best meets your practice laboratory.
Running the UDP socket server on Linux.
On a machine with Linux, we can open the terminal and type the command below. And then paste the server code you copied above.
nano serverudp.py
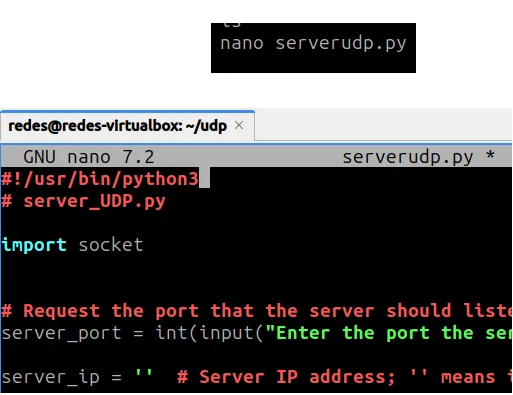
After pasting the code and saving, we will run the “server.py“. For this, let’s type the command below.
python3 serverudp.py
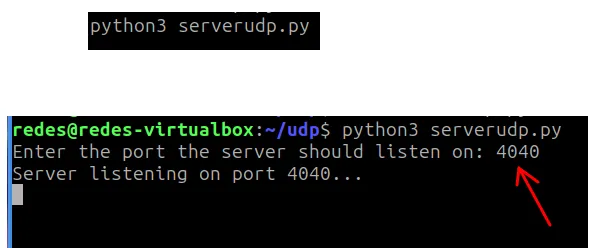
We can see that the server is listening at port 4040 and waiting for connections.
Explaining the UDP socket server code.
Now let’s explain the UDP socket server code from our chat.
Initially we will ask what door the server will hear. My suggestion is to use high doors such as 4040.
# Request the port that the server should listen on
server_port = int(input(“Enter the port the server should listen on: “))
server_ip = ” # Server IP address; ” means it will listen on all interfaces
Then we will create the UDP socket that will be of type IPv4 ? “AF_INET” and will be UDP – “SOCK_DGRAM“.
# Create the UDP socket
udp = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
Now, let’s set up the IP and the port we want to monitor. In this case, we are considering that the IP will be “0.0.0.0“, that is, the server will be listening on the specified port on all the IPs available on the machine.
Next we will store connection data in two variables. The “server_ip” variable stores the server’s IP and the “server_port” variable presents the port that the server will listen.
# Define the server address (IP and port)
server_address = (server_ip, server_port)
udp.bind(server_address) # Bind the IP and port to start listening
Next, let’s see the loop that awaits incoming messages that are harvested with socket.
- recvfrom(1024) = reads up to 1024 bytes of the message sent by the client via the socket.
- received_message.decode(“utf8”) = decoded from bytes to string the received message.
while True:
# Receive data from the client (data, address)
received_message, client_address = udp.recvfrom(1024)
# Decode the message from bytes to string
received_message = received_message.decode(“utf8”)
Finally we have the end of socket when the user presses “CTRL+c”.
udp.close()
Explaining the UDP chat client.
Next, we will show the client code of the chat in python and then how to run it.
#!/usr/bin/python3
# client_UDP.py
import socket
def main():
# Request the Server's IP and Port
server_IP = input("Enter the server's IP address: ")
server_port = int(input("Enter the server's port: "))
# Create the UDP socket
udp = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# Define the destination (IP and port)
DESTINATION = (server_IP, server_port)
try:
print("Type your messages. To exit, type 'exit'.")
while True:
# Get the user's message
message = input("Enter the message to be sent: ")
if message.lower() == 'exit':
print("Closing the client.")
break
# Send the message to the destination
udp.sendto(bytes(message, "utf8"), DESTINATION)
print("Message sent successfully!")
except Exception as e:
print(f"Error during communication: {e}")
finally:
# Close the socket
udp.close()
if __name__ == "__main__":
main()
Running the UDP socket client on Windows.
Let’s open an editor like the Notepad and we’re going to paste the code we copy above. Then we’ll click save.
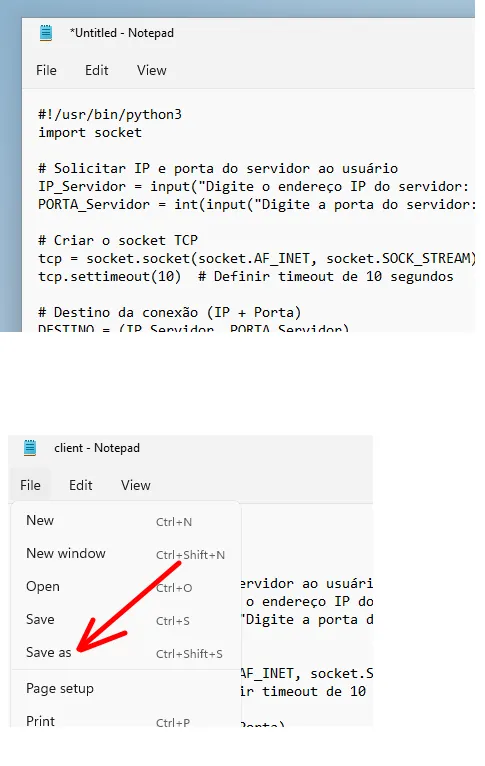
At that point, we will choose where we will create the file and the name. In this case we will use the name “clientudp.py“.
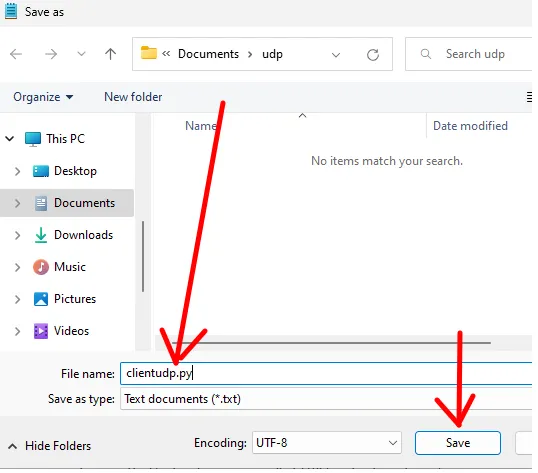
Now, we go to the directory where you saved the “clientudp.py” and we can double click on the client to run it.
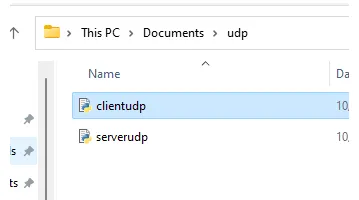
When running the “clientudp.py” we will indicate the server’s IP. In this case, we are using the loopback address the “127.0.0.1” but you can choose the IP of a machine from your network that is running the “serverudp.py“.
Next, let’s type the door that the server is listening to, which in this example was port 4040.
Then we send a “hello” message.
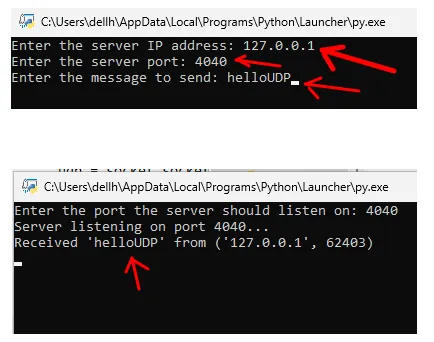
We can see in the figure above that the server received the message “hello” that the client sent.
Running the UDP socket client on Linux.
To run the client on Linux, we will open a terminal and use an editor. In our case we are using the “nano” editor. So let’s type the command below to create and edit the file “clientudp.py“.
nano clientudp.py
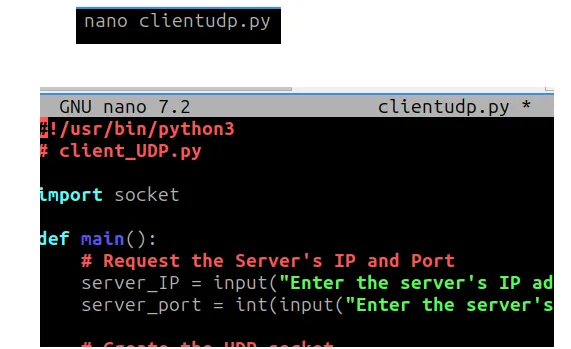
After opening the editor, we will paste the client code that we copy above and then save.
Now, let’s run the “clientudp.py” using the command below.
python3 clientudp.py
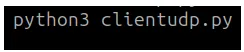
When running the “clientudp.py” we will indicate the server’s IP. In this case, we are using the loopback address the “127.0.0.1” but you can choose the IP of a machine from your network that is running the “serverudp.py“.
Next, let’s type the door that the server is listening to, which in this example was port 4040.
Now, let’s type “hello” in the client.
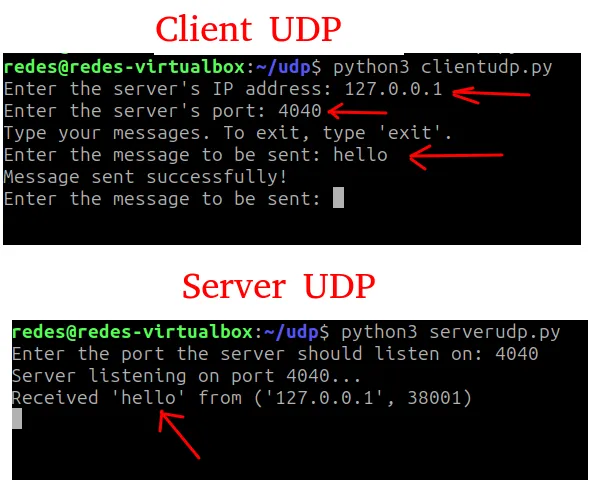
We can see that the server “serverudp.py” received the “hello” server from the client.
Explaining the UDP socket client code.
Initially we are asking the user to indicate the IP and the port that the client will connect. In this case, we will insert the IP of the server and the port that the server is listening to.
# Request the Server’s IP and Port
server_IP = input(“Enter the server’s IP address: “)
server_port = int(input(“Enter the server’s port: “))
Agora, vamos criar o socket UDP .
# Create the UDP socket
udp = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
The first line creates a socket for client-server communication using:
- AF_INET = IPv4 as an address family.
- SOCK_DGRAM = UDP as a communication protocol, which means that communication will not be connected-oriented, will not be reliable, and without flow control.
Next, we will create the variable that will be used as the UDP communication destination. In this case we have the combination of the IP and server port.
# Define the destination (IP and port)
DESTINATION = (server_IP, server_port)
Next, we will see the snippet of the code that allows the user to send multiple messages to the server until he decides to close the program.
# Get the user’s message
message = input(“Enter the message to be sent: “)
Next, we go to the function that will connect to the server “udp.sendto“. This function takes as parameter the set (IP + server port) that we have previously defined as “DESTINATION“.
# Send the message to the destination
udp.sendto(bytes(message, “utf8”), DESTINATION)
- input() = Solicita ao usuário que digite uma mensagem.
- udp.sendto() = função para enviar a mensagem.
- bytes(Mensagem, “utf8”) = The message is converted to bytes to be sent over the network.
Then we will have the function to check if there was error in socket “except Exception” and finally, we will have the function to finish the socket with “udp.close()“.
except Exception as e:
print(f”Error during communication: {e}”)
finally:
# Close the socket
udp.close()
Read more:
Python get metadata from images and pdfs
How to connect mysql using python
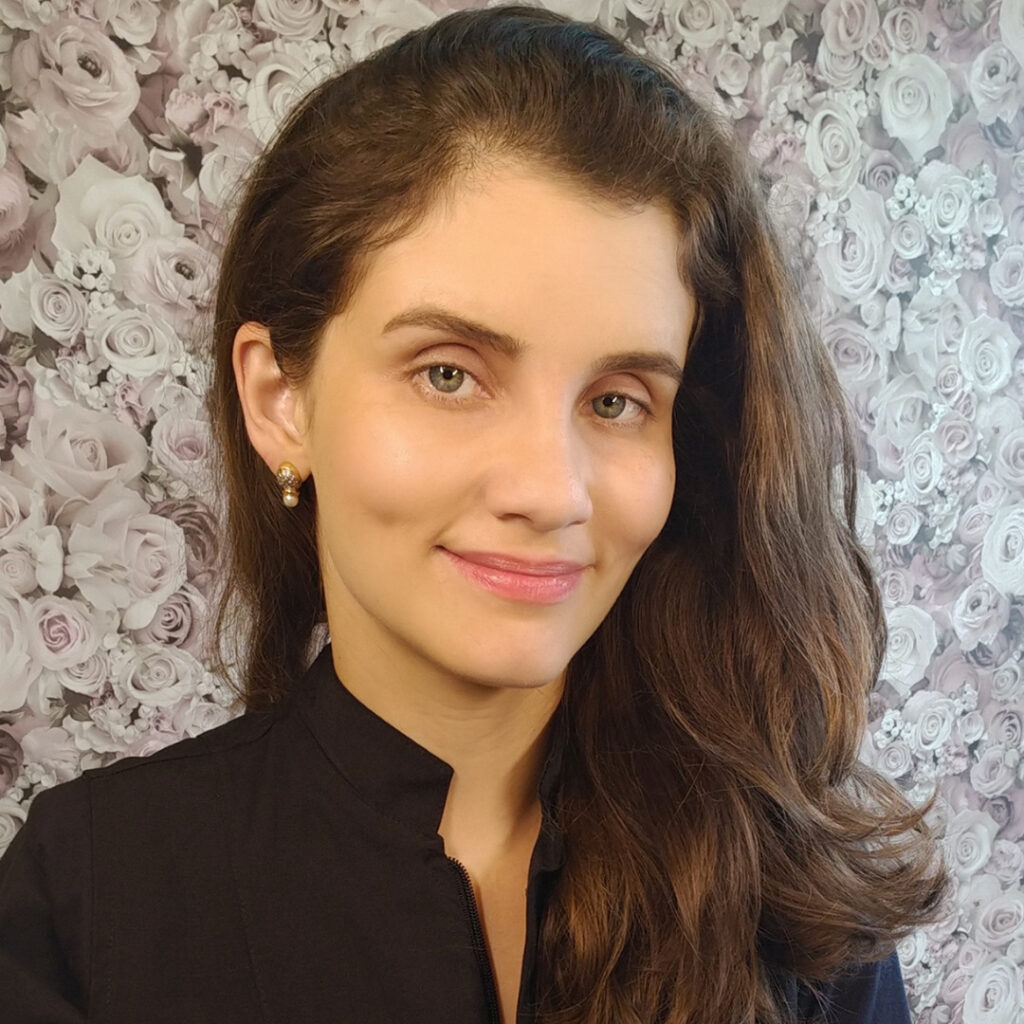
Juliana Mascarenhas
Data Scientist and Master in Computer Modeling by LNCC.
Computer Engineer